Test-Driven Development for C Training
This training course helps you build knowledge, understanding and skill in the engineering practices needed to build great C code. You learn how to build flexible and modular software with very few defects, software that can have a long useful life.
Among other things, you'll learn the difference between Debug-Later Programming (the most popular programming technique on the planet) and Test-Driven Development.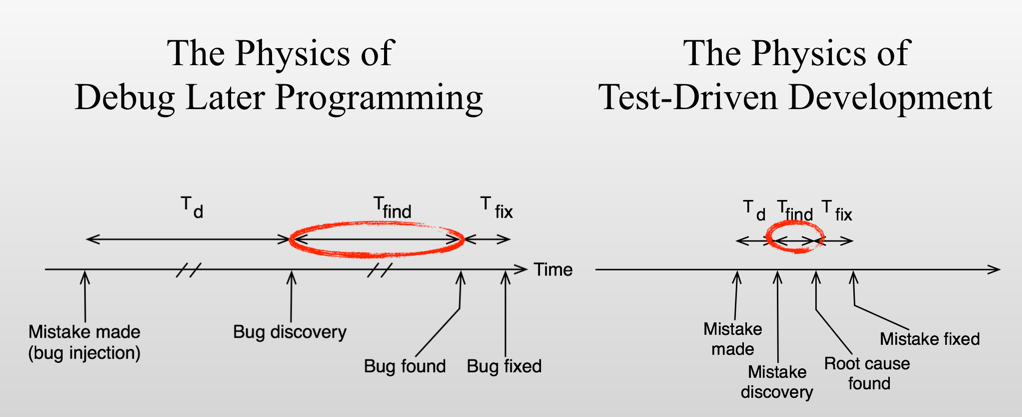
Learning Model
We learn new skills by doing, not just reading, listening, and thinking. Engineers are not so interested in changing how they work if the change does not help solve some identified problem. We’ve found this learning cycle to be invaluable to attendees of our courses.
- Present a problem -- problems motivate change
- Present a potential solution -- the idea that may help
- Demonstrate part of the solution -- remove ambiguity
- Participant does an exercise -- learn by doing
- Experience debrief -- identify and discuss both positive and negative reactions
In this course, we repeat this learning cycle, growing skills with each iteration. Our goal is to build new skills on top of the attendees' existing skills.
Course Length Options
- 2 days - Hands-on classroom only. This course can be delivered live-via-the-web
- 4 days with Legacy Code Workshop (Recommended for existing code)
- 4 days with Walking Skeleton Workshop (Recommended for greenfield)
- For smaller groups a one day workshop is available.
Audience
- Software Developers
- Technical team leaders
- Managers that want to know more about the technology they manage
Course Outline
- Why Test Driven Development?
- What is Test Driven Development?
- The Microcycle
- Exercise
- Debrief
- Object Oriented Principles Applied to C
- Designing to interfaces
- Information hiding
- Substitutability
- Spying
- Faking the Time
- Link-time Fake
- Exercise
- Debrief
- Tests as Detailed Documentation
- Four-Phase Test Pattern
- Given, When, Then
- Arrange, Act Assert
- Test Smells
- Duplication in Tests
- Taxonomy of Test-Doubles
- Choosing Real or Test-Double
- The Problem Solved by Mock Objects
- Specifying and Satisfying Expectations
- Exercise
- Debrief
- Refactoring Defined
- Critical to a Healthy Business
- Critical Skills
- Code Smells
- Envisioning
- Transforming
- TDD's Positive Influence on Design
- Legacy Code Mindset
- The Cost of Doing Business
- Where and How to Add Test and Refactor
- Boy Scout Rule -- leave the campsite better than you found it
- Incremental Improvement
- Legacy Change Algorithm
- Crash to Pass Algorithm
- Wrap up Discussion
- Optional Workshop for your Product Begins for on-site courses.
Test Driven Development
Module Objective: Software developers make mistakes. Mistakes undetected become defects with potentially huge costs. In this section, attendees can learn the motivations behind TDD and how the short test-driven cycles may help prevent defects.
During the debrief, we explore what people liked about TDD and what concerns them. Most attendees like the experience, but also have a serious concerns or two. It is important to bring the concerns into the open. This is a rare opportunity to reflect on how we work and to envision how TDD may help us improve.
Your Software Development Environment
Module Objective: Look at what is special about your programming environment, and some of the current industry-standard practice inefficiencies. We hope to show that TDD is well suited for improving the product quality, as well as reducing some of the frustrations and waste when testing code in your environment.
TDD and Collaborating Modules - testing the code in the middle
Module Objective: The most valuable code you have (the code with the potential longest life) has dependencies. This code embodies what makes your product special. Automated tests help preserve your investment, allowing changes that have fewer unwanted side effects (defects!). We'll look at principles and techniques to guide developers in creating modular, testable, and tested embedded software. We'll introduce Spies and Fakes. You'll see how Spies and Fakes can fully exercise the code under test.
Keeping Tests Clean
Module Objective: Tests have many valuable uses. One overlooked value of tests is that they are documentation. Tests provide an executable specification of the code under test. This document shows how the code is supposed to be used and how it is supposed to work. This document is unambiguous; it's code! This document warns you whenever the code differs from the specification. To get the most value from tests as documentation, tests must be written to be read and understood.
Test-Doubles
Module Objectives: There is more to test-stubs than the Spy and the Fake. We'll look at an overview of Test-Doubles and when to use them.
Test-Driving With Mock Objects
Module Objective: Mocking is an important tool for checking that the code under test is interacting properly with its dependencies.
Refactoring -- Overview
Module Objective: As requirements and users' needs change, code must change. Design is so important, we don't just do design once at the beginning of the development effort. Design is a continuous process. We accept that changing design is dangerous, and the tests created by the test-driven developer provide a safety-net to lock in code behavior as your product design evolves.
Refactoring (changing the structure of code, without changing its behavior) is a step in the TDD microcycle used when we need to clean up a mess. We'll also refactor code so that a new feature can be dropped in. This module provides and overview of the mindset and the critical skills needed to evolve designs for a long useful life.
For more depth in design and refactoring, you can follow our TDD course with SOLID Design and Refactoring for C
Working with Legacy Code -- Overview
Module Objective: Now we face the reality that we have code that was not created with TDD. We've seen how TDD can help guide creating tested, modular and loosely coupled software. The reality: our code has problems. We don't have time to stop all feature development and retrofit our code base with tests. We'll look at the pragmatic approach to incrementally improving your valuable code base.
This course will get you and your team well on the way to applying TDD in your C development efforts.
Latest News
Conference Video - Deep Stack – Tracer Bullets from ADC to Browser
A blank page can be very intimidating, even for a Test-driven developer. Where do we start? Write a test, right? Not always.
more...Podcast on Agile Amped
Here is a short interview with James about TDD and embedded software from the deliver:Agile conference last spring.
more...Programming Research -- Please Participate
Do you have some time to do a simple programming problem in C or C++ for my research?
more...Clean Coders IoT Case Study
My long-time good friend (Uncle) Bob Martin and I have fun programming together firing tracer bullets for distributed water pressure measurement system.
more...Books
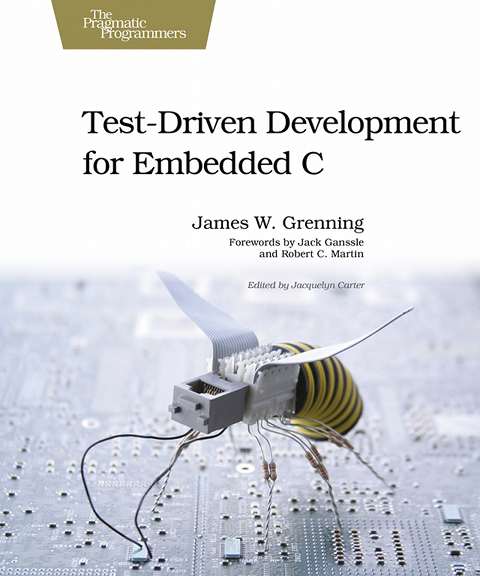
James is the author of Test-Driven Development for Embedded C.
Have you read Test-Driven Development for Embedded C? Please write a review at
Amazon
or
Good Reads
.