Programming Research -- Please Participate
I want to see how C and C++ programmers solve a simple programming problem, CircularBuffer. There are no tools to set up. You will work on my cyber-dojo system. It has a build environment and a simple IDE.
Here are some of the instructions you will find when you do the exercise
Objective --------- Write a CircularBuffer and make sure it works. Requirements ------------ * A CircularBuffer is sized during create. * It stores integers. * It is FIFO. * It can report its total capacity * It can report if it is empty * It can report if it is full * Putting to a full CircularBuffer * loses no prior values * returns false * Getting from an empty CircularBuffer returns a default value provided during create function. For this exercise, do not worry about: -------------------------------------- * Null pointers * Concurrency * Memory allocation failures CircularBuffer Diagram ---------------------- www.wingman-sw.com/files/cyber-dojo/CircularBuffer.pdf Starting point -------------- The CircularBuffer interface is defined in CircularBuffer.h. You must develop your implementation to that interface. The architects said so.
Tweet
If you are interested, please send an email to 'research AT wingman-sw DOT com'. I will send you an invitation to participate.
Let me know if you usually use a unit test framework. I'll have one there ready for you to use. If you do not use a unit test framework, no worries. You'll test the code as you see fit.
Published: January 05, 2018
Latest News
Conference Video - Deep Stack – Tracer Bullets from ADC to Browser
A blank page can be very intimidating, even for a Test-driven developer. Where do we start? Write a test, right? Not always.
more...Podcast on Agile Amped
Here is a short interview with James about TDD and embedded software from the deliver:Agile conference last spring.
more...Programming Research -- Please Participate
Do you have some time to do a simple programming problem in C or C++ for my research?
more...Clean Coders IoT Case Study
My long-time good friend (Uncle) Bob Martin and I have fun programming together firing tracer bullets for distributed water pressure measurement system.
more...Books
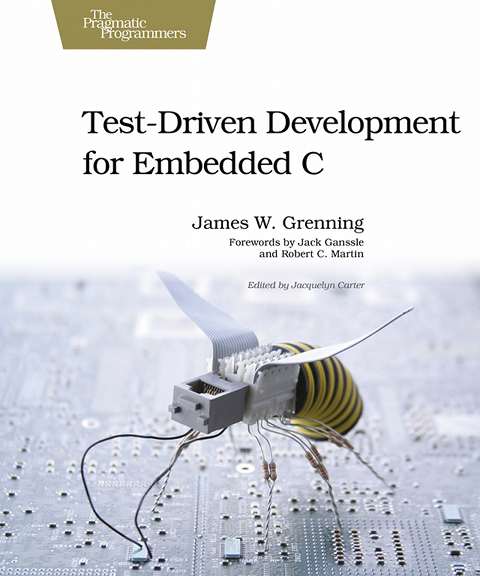
James is the author of Test-Driven Development for Embedded C.
Have you read Test-Driven Development for Embedded C? Please write a review at
Amazon
or
Good Reads
.